django view example and render function
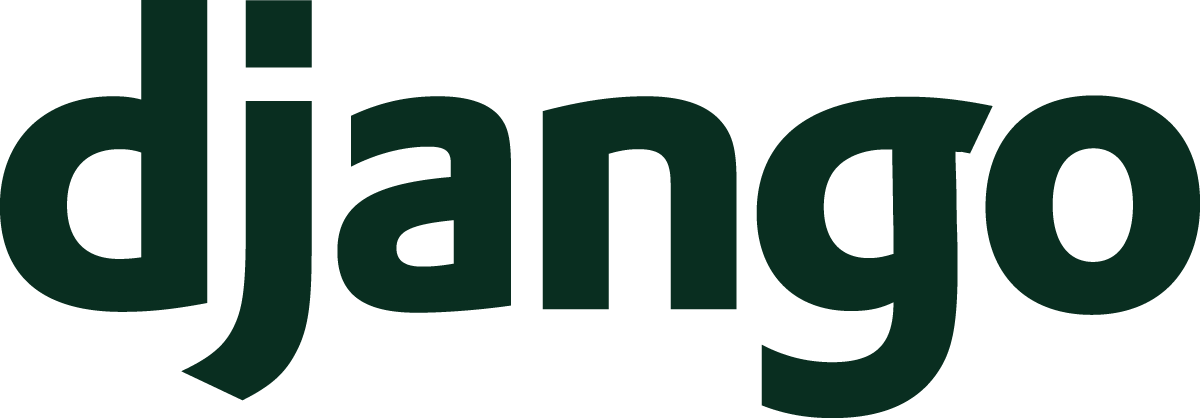
def employee_list(request):
employees = Employee.objects.all()
return render(request, 'employee_list.html', {'employees': employees})
In the given view, here are the steps explained simply:
- The
employee_list
function is defined, which takes arequest
parameter representing the HTTP request made to the server. - Inside the function, all the
Employee
objects are retrieved from the database using theEmployee.objects.all()
query. This fetches all the employee records. - The retrieved employees are then passed as a context variable called
'employees'
to therender
function, along with the'employee_list.html'
template name. - The
render
function combines the provided template with the context data and generates an HTTP response. - The generated HTTP response, containing the rendered HTML content, is sent back to the client's web browser.
In summary, the view retrieves all employee records from the database, sends them to the 'employee_list.html'
template, and returns the resulting HTML response to the client.
The render
function is a utility function provided by Django that combines a provided template with the context data and generates an HTTP response to be sent back to the client.
The most commonly used parameters when calling the render
function are:
request
(required): Therequest
object representing the incoming HTTP request.template_name
(required): The name of the template file to be rendered. This can be a string specifying the template's filename or a list of template names, in which case Django will use the first template that exists.context
(optional): A dictionary containing the context data to be passed to the template. The keys in the dictionary represent the variable names accessible in the template, and the corresponding values are the data to be displayed or processed in the template.content_type
(optional): The MIME type of the response content. By default, it is set to"text/html"
.status
(optional): The HTTP status code to be set in the response. The default is200
(OK).using
(optional): The name of the database connection to use for the rendering process. This is relevant when working with multiple databases.
Here's an example usage of the render
function:
pythonfrom django.shortcuts import
renderdef my_view(request
):
data = {'name': 'John'
,'age': 30
,
}return render(request, 'my_template.html'
, context=data)
In this example, the render
function takes the request
object, the template name 'my_template.html'
, and the context data data
. It combines the template with the provided context and generates an HTTP response with the rendered content.
Help documents
To see the details of the render()
function in Django, you can use the help()
function like this:
pythonimport
django.shortcutshelp
(django.shortcuts.render)
This will display the detailed information about the render()
function, including the parameters it accepts, their types, and their descriptions. It will also provide information about any default values and possible exceptions that can be raised.
Additionally, you can also check the Django documentation or the source code of the render()
function to get more detailed information about its usage and requirements.
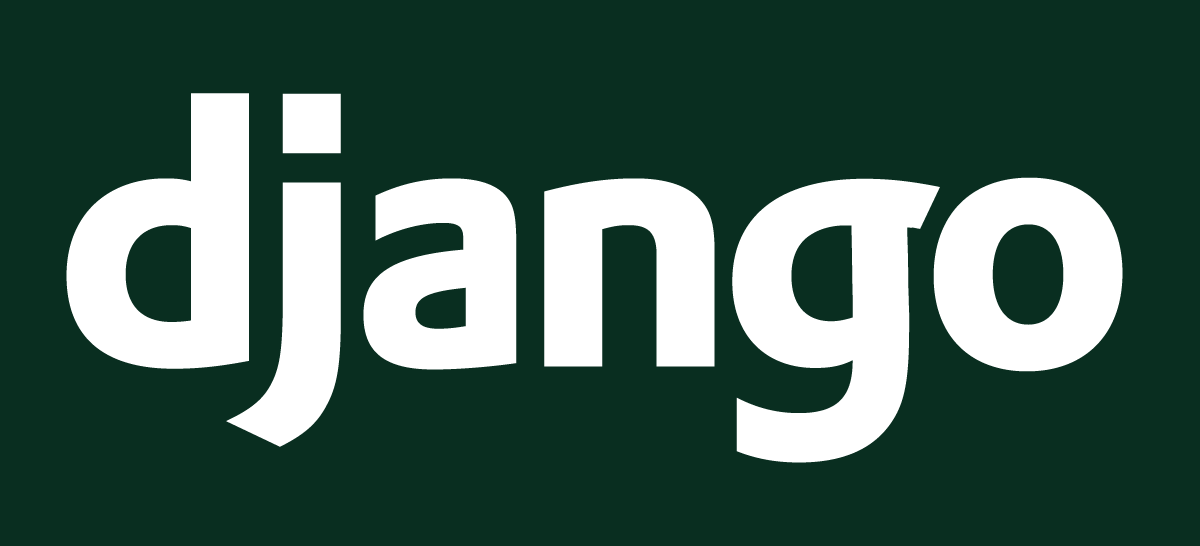