Django Listview compared to models.model
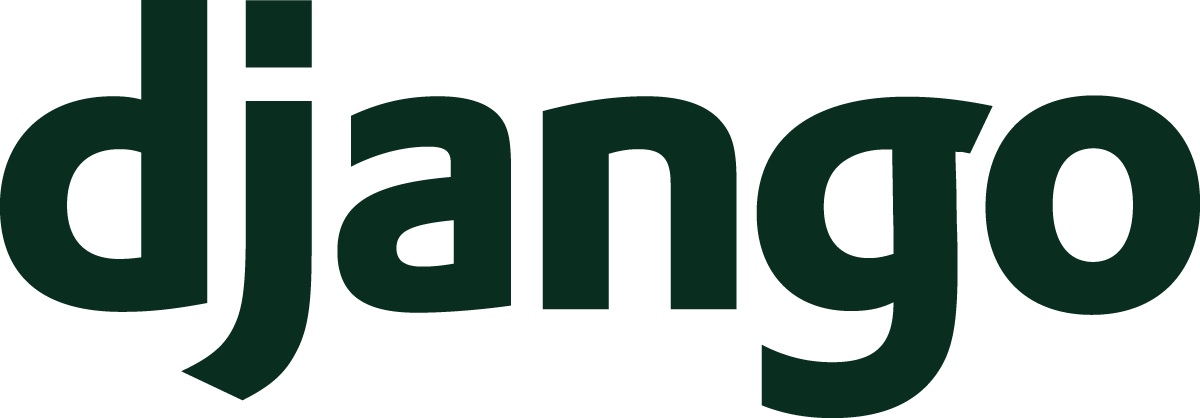
In Django, ListView
and models.Model
are different concepts that serve different purposes.
models.Model
is a base class provided by Django for creating database models. When you define a class that inherits from models.Model
, you are creating a database table and specifying the fields and behaviors of that table. Each instance of the class represents a row in the table, and you can perform database operations (e.g., saving, updating, deleting) using the model.
On the other hand, ListView
is a class-based view provided by Django that is used to display a list of objects. It is typically used in combination with a model to retrieve a queryset of objects and render them in a template. ListView
takes care of retrieving the objects, paginating the results if necessary, and rendering the list in the template.
To use ListView
, you need to define a model (inheriting from models.Model
) and a subclass of ListView
that specifies the model and template to use. The ListView
will handle retrieving the objects from the database and rendering them in the specified template.
Here's an example that illustrates the usage of ListView
:
pythonfrom django.views.generic import
ListViewfrom .models import
YourModelclass YourListView(ListView
):
model = YourModeltemplate_name = 'your_template.html'
In this example, YourModel
is a subclass of models.Model
that represents your database table. YourListView
is a subclass of ListView
that specifies the model to use (YourModel
) and the template to render (your_template.html
).
When you access the URL associated with YourListView
, Django will automatically retrieve the objects from the database using the YourModel
queryset and render them in the specified template.
Potential Benefits
- Code reusability:
ListView
is a generic view provided by Django that encapsulates common functionality for displaying lists of objects. By usingListView
, you can leverage the built-in functionality and reduce the amount of custom code you need to write. - Consistency:
ListView
follows a consistent pattern for retrieving objects from the database, paginating the results, and rendering them in a template. This helps maintain a consistent structure across your views and improves code readability. - Pagination:
ListView
includes built-in pagination support, allowing you to easily handle large lists of objects by splitting them into pages. It provides pagination controls in the template, such as previous/next links and page numbers. - Flexibility:
ListView
provides various hooks and attributes that allow you to customize its behavior according to your needs. You can override methods likeget_queryset()
to modify the object retrieval logic, orget_context_data()
to add additional context variables to the template. - Integration with templates:
ListView
works seamlessly with Django's template engine. It automatically passes the retrieved objects to the template context, making it easy to iterate over them and display the data.
Potential downsides
- Less flexibility:
ListView
is a generic view that provides a predefined set of functionality. If your requirements are complex or deviate significantly from the default behavior, you may find it more challenging to customize the view to fit your specific needs. In such cases, writing a custom view may offer more flexibility. - Learning curve: Understanding how to utilize and customize
ListView
effectively requires some familiarity with Django's class-based views and the concepts they employ. If you're new to Django or class-based views, there may be a learning curve involved in grasping the underlying concepts and effectively usingListView
. - Performance considerations:
ListView
retrieves all objects from the database that match the provided queryset, and by default, it paginates the results. This means that if you have a large number of objects, it could impact the performance and load times of your view. In such cases, you may need to optimize the query or implement additional caching mechanisms. - Limited control over HTML structure: The HTML structure and layout of the rendered list are determined by the default template used by
ListView
. While you can override the template to some extent, you may have limited control over the exact HTML structure and styling of the rendered list. This can be a limitation if you have specific design requirements. - Potential code complexity: As your project grows and your view requirements become more intricate, using a generic view like
ListView
might lead to complex subclassing, overriding methods, and managing various view-related functionalities. This complexity can make the code harder to understand and maintain in the long run.
It's important to weigh these potential downsides against the benefits and consider whether ListView
aligns well with your specific project requirements and development approach. In some cases, a custom view tailored to your needs may be a more suitable option.
More about listview
ListView
is a class-based view in Django. It is a pre-defined class-based view provided by Django that is used for displaying a list of objects from a database query. It inherits from the django.views.generic.list.ListView
class.
When you use ListView
in your Django application, you need to specify the model that the view should fetch data from using the model
attribute. Additionally, you can customize the behavior of the ListView
by overriding its methods or specifying other attributes like template_name
to define the template used for rendering the list of objects.
Here's an example of how you can use ListView
in your Django views:
pythonfrom django.views.generic import
ListViewfrom .models import
MyModelclass MyModelListView(ListView
):
model = MyModeltemplate_name = 'myapp/mymodel_list.html'
context_object_name = 'mymodels'
In this example, MyModelListView
is a subclass of ListView
, and it specifies the MyModel
model as the source of data for the list view. The template_name
attribute specifies the template used to render the list of objects, and context_object_name
sets the name of the variable used to access the list of objects in the template.
By using ListView
, you inherit the default behavior and methods provided by the class-based view, such as retrieving the list of objects from the database, paginating the results, and rendering the template with the list of objects.
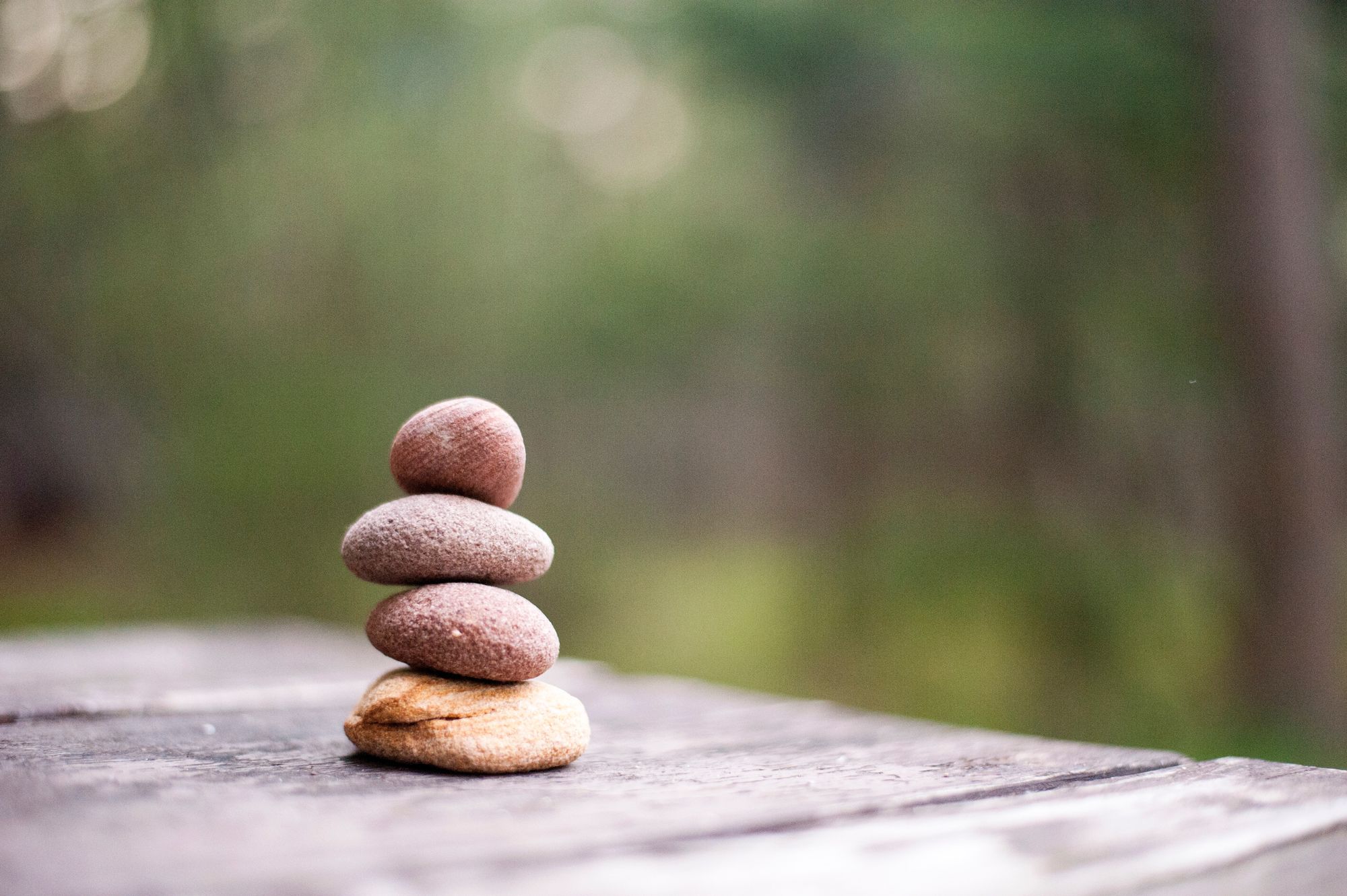