DJANGO what is the difference between class-based view and function-based view
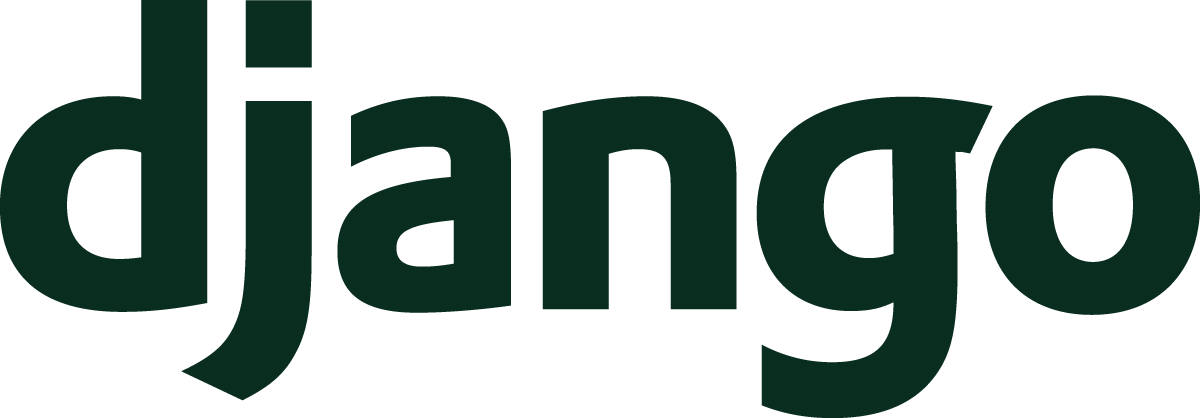
The main difference between class-based views (CBVs) and function-based views (FBVs) in Django is the way they are implemented and organized.
Function-based views (FBVs) are defined as Python functions that accept a request object and return a response. They are typically simpler and more straightforward to write. FBVs are well-suited for simple views that don't require a lot of custom logic or complex behavior.
On the other hand, class-based views (CBVs) are implemented as Python classes that inherit from Django's base view classes. CBVs provide a more structured and object-oriented approach to handling views. They offer a higher level of abstraction and encapsulation, allowing you to define reusable view mixins, leverage class inheritance, and override methods for different stages of the view processing (e.g., get()
, post()
, etc.). CBVs are well-suited for complex views that require more advanced functionality, such as handling different HTTP methods, form processing, or interacting with models.
Here are some key differences between CBVs and FBVs:
- Code organization: CBVs provide a structured way to organize view-related code by encapsulating it within class-based views. This can lead to more maintainable and reusable code.
- Inheritance and mixins: CBVs allow you to leverage class inheritance and use mixins to compose views with reusable functionality. This can simplify code reuse and promote DRY (Don't Repeat Yourself) principles.
- Method-based handling: CBVs define different methods (such as
get()
,post()
, etc.) that correspond to different HTTP methods. This makes it easier to handle different types of requests within a single view class. - Mixin-based customization: CBVs allow you to mix in additional functionality using mixins, which are reusable classes that can be combined with view classes to provide additional behavior.
- Class-based decorators: CBVs allow decorators to be applied directly to the view class, making it easier to apply custom logic or modifications to the entire view.
- Default behavior: CBVs come with built-in default behavior and methods for handling common tasks like rendering templates, handling form validation, and processing HTTP requests. This can save you time and effort compared to writing all the logic from scratch in an FBV.
Overall, CBVs offer more flexibility, code organization, and reusability compared to FBVs. However, FBVs can still be a good choice for simple views or situations where the additional complexity and abstraction of CBVs are not necessary. It ultimately depends on the complexity and requirements of your project.
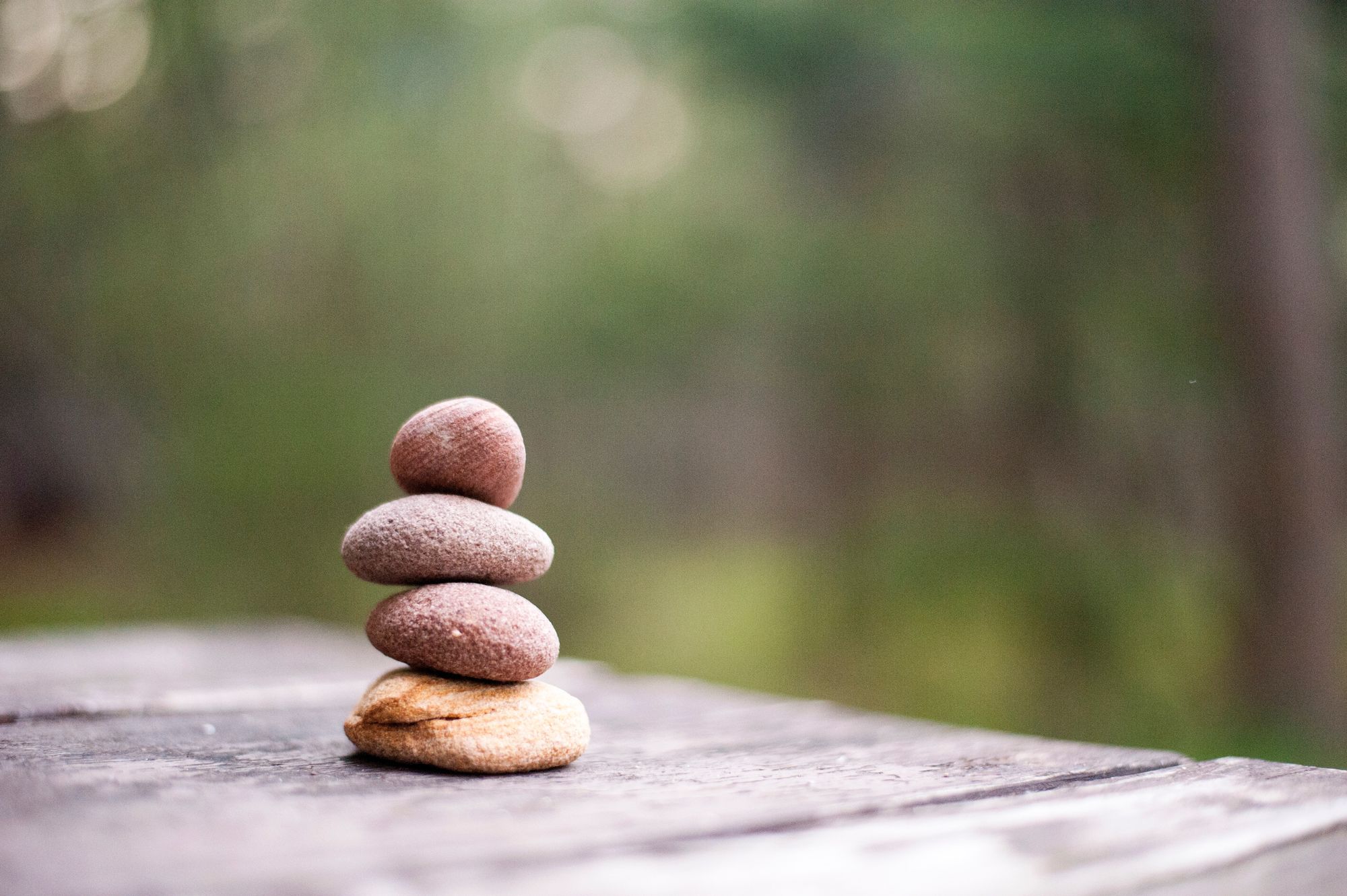